Image Reconstruction
[1]:
# This cells setups the environment when executed in Google Colab.
try:
import google.colab
!curl -s https://raw.githubusercontent.com/ibs-lab/cedalion/dev/scripts/colab_setup.py -o colab_setup.py
# Select branch with --branch "branch name" (default is "dev")
%run colab_setup.py
except ImportError:
pass
Notebook configuration
Decide for an example with a sparse probe or a high density probe for DOT. The notebook will load example data accordingly.
Also specify, if precomputed results of the photon propagation should be used and if the 3D visualizations should be interactive.
[2]:
# choose between two datasets
DATASET = "fingertappingDOT" # high-density montage
#DATASET = "fingertapping" # sparse montage
# choose a head model
HEAD_MODEL = "colin27"
# HEAD_MODEL = "icbm152"
# choose between the monte
FORWARD_MODEL = "MCX" # photon monte carlo
#FORWARD_MODEL = "NIRFASTER" # finite element method - NOTE, you must have NIRFASTer installed via runnning <$ bash install_nirfaster.sh CPU # or GPU> from a within your cedalion root directory.
# set this flag to False to actual compute the forward model results
PRECOMPUTED_FLUENCE = True
# set this flag to True to enable interactive 3D plots
INTERACTIVE_PLOTS = False
%load_ext autoreload
%autoreload 2
[3]:
import pyvista as pv
if INTERACTIVE_PLOTS:
pv.set_jupyter_backend('server')
else:
pv.set_jupyter_backend('static')
import os
import time
import warnings
from pathlib import Path
from tempfile import TemporaryDirectory
import matplotlib.pyplot as p
import numpy as np
import xarray as xr
from IPython.display import Image
from pint.errors import UnitStrippedWarning
import cedalion
import cedalion.dataclasses as cdc
import cedalion.datasets
import cedalion.geometry.registration
import cedalion.geometry.segmentation
import cedalion.imagereco.forward_model as fw
import cedalion.imagereco.tissue_properties
import cedalion.io
import cedalion.plots
import cedalion.sigproc.quality as quality
import cedalion.vis.plot_sensitivity_matrix
from cedalion import units
from cedalion.imagereco.solver import pseudo_inverse_stacked
from cedalion.io.forward_model import FluenceFile, load_Adot
xr.set_options(display_expand_data=False);
Working Directory
In this notebook tHe output of the fluence and sensitivity calculations are stored in a temporary directory. This will be deleted when the notebook ends.
[4]:
temporary_directory = TemporaryDirectory()
working_directory = Path(temporary_directory.name)
Load a DOT finger-tapping dataset
For this demo we load an example finger-tapping recording through cedalion.datasets.get_fingertapping
. The file contains a single NIRS element with one block of raw amplitude data.
[5]:
if DATASET == "fingertappingDOT":
rec = cedalion.datasets.get_fingertappingDOT()
elif DATASET == "fingertapping":
rec = cedalion.datasets.get_fingertapping()
else:
raise ValueError("unknown dataset")
The location of the probes is obtained from the snirf metadata (i.e. /nirs0/probe/)
Note that units (‘m’) are adopted and the coordinate system is named ‘digitized’.
[6]:
geo3d_meas = rec.geo3d
display(geo3d_meas)
<xarray.DataArray (label: 346, digitized: 3)> Size: 8kB [mm] -77.82 15.68 23.17 -61.91 21.23 56.49 ... 14.23 -38.28 81.95 -0.678 -37.03 Coordinates: type (label) object 3kB PointType.SOURCE ... PointType.LANDMARK * label (label) <U6 8kB 'S1' 'S2' 'S3' 'S4' ... 'FFT10h' 'FT10h' 'FTT10h' Dimensions without coordinates: digitized
[7]:
cedalion.plots.plot_montage3D(rec["amp"], geo3d_meas)
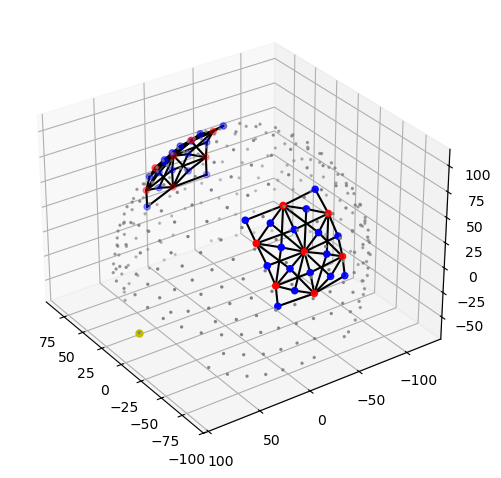
The measurement list is a pandas.DataFrame
that describes which source detector pairs form channels.
[8]:
meas_list = rec._measurement_lists["amp"]
display(meas_list.head(5))
sourceIndex | detectorIndex | wavelengthIndex | wavelengthActual | wavelengthEmissionActual | dataType | dataUnit | dataTypeLabel | dataTypeIndex | sourcePower | detectorGain | moduleIndex | sourceModuleIndex | detectorModuleIndex | channel | source | detector | wavelength | chromo | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 1 | 1 | 1 | None | None | 1 | None | raw-DC | 1 | None | None | None | None | None | S1D1 | S1 | D1 | 760.0 | None |
1 | 1 | 2 | 1 | None | None | 1 | None | raw-DC | 1 | None | None | None | None | None | S1D2 | S1 | D2 | 760.0 | None |
2 | 1 | 4 | 1 | None | None | 1 | None | raw-DC | 1 | None | None | None | None | None | S1D4 | S1 | D4 | 760.0 | None |
3 | 1 | 5 | 1 | None | None | 1 | None | raw-DC | 1 | None | None | None | None | None | S1D5 | S1 | D5 | 760.0 | None |
4 | 1 | 6 | 1 | None | None | 1 | None | raw-DC | 1 | None | None | None | None | None | S1D6 | S1 | D6 | 760.0 | None |
Event/stimulus information is also stored in a pandas.DataFrame
. Here events are given more descriptive names:
[9]:
if DATASET == "fingertappingDOT":
rec.stim.cd.rename_events( {
"1": "Control",
"2": "FTapping/Left",
"3": "FTapping/Right",
"4": "BallSqueezing/Left",
"5": "BallSqueezing/Right"
} )
elif DATASET == "fingertapping":
rec.stim.cd.rename_events( {
"1.0": "Control",
"2.0": "FTapping/Left",
"3.0": "FTapping/Right"
} )
display(rec.stim.groupby("trial_type")[["onset"]].count())
onset | |
---|---|
trial_type | |
BallSqueezing/Left | 17 |
BallSqueezing/Right | 16 |
Control | 65 |
FTapping/Left | 16 |
FTapping/Right | 16 |
Perform pruning, conversion to OD and bandpass filtering
(for this demo select 20 seconds after a trial starts at t=117s and transform raw amplitudes to optical density)
Perform SNR quality check and pruning and then transform CW raw amplitudes to optical density
[10]:
## Prune with SNR threshold
snr_thresh = 10 # dB
snr, rec.masks["snr_mask"] = quality.snr(rec["amp"], snr_thresh)
# prune channels using the masks and the operator "all", which will keep only channels that pass all three metrics
rec["amp_pruned"], drop_list = quality.prune_ch(rec["amp"], [rec.masks["snr_mask"]], "all")
print(drop_list)
# Convert to OD
rec["od"] = cedalion.nirs.int2od(rec["amp_pruned"])
# bandpass filter the data
rec["od_freqfiltered"] = rec["od"].cd.freq_filter(fmin=0.01, fmax=0.5, butter_order=4)
[]
Calculate block averages in optical density
[11]:
# segment data into epochs
epochs = rec["od_freqfiltered"].cd.to_epochs(
rec.stim, # stimulus dataframe
["FTapping/Left", "FTapping/Right"], # select events, discard the others
before=5 * cedalion.units.s, # seconds before stimulus
after=30 * cedalion.units.s, # seconds after stimulus
)
# calculate baseline
baseline = epochs.sel(reltime=(epochs.reltime < 0)).mean("reltime")
# subtract baseline
epochs_blcorrected = epochs - baseline
# group trials by trial_type. For each group individually average the epoch dimension
blockaverage = epochs_blcorrected.groupby("trial_type").mean("epoch")
# Plot block averages. Please ignore errors if the plot is too small in the HD case
noPlts2 = int(np.ceil(np.sqrt(len(blockaverage.channel))))
f,ax = p.subplots(noPlts2,noPlts2, figsize=(12,10))
ax = ax.flatten()
for i_ch, ch in enumerate(blockaverage.channel):
for ls, trial_type in zip(["-", "--"], blockaverage.trial_type):
ax[i_ch].plot(blockaverage.reltime, blockaverage.sel(wavelength=760, trial_type=trial_type, channel=ch), "r", lw=2, ls=ls)
ax[i_ch].plot(blockaverage.reltime, blockaverage.sel(wavelength=850, trial_type=trial_type, channel=ch), "b", lw=2, ls=ls)
ax[i_ch].grid(1)
ax[i_ch].set_title(ch.values)
ax[i_ch].set_ylim(-.02, .02)
ax[i_ch].set_axis_off()
ax[i_ch].axhline(0, c="k")
ax[i_ch].axvline(0, c="k")
p.suptitle("760nm: r | 850nm: b | left: - | right: --")
p.tight_layout()
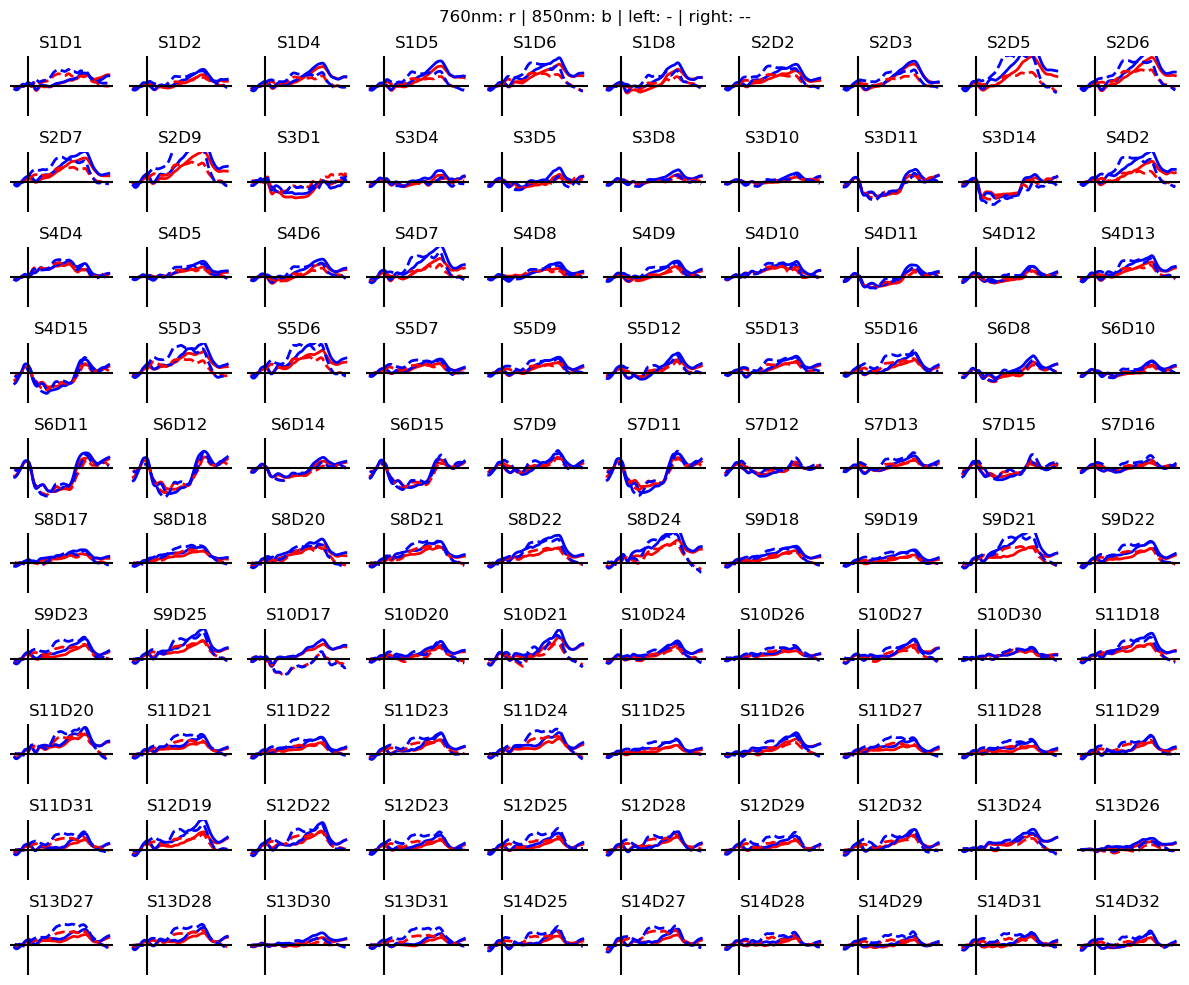
Load segmented MRI scan
For this example use a segmentation of the Colin27 average brain.
[12]:
if HEAD_MODEL == "colin27":
SEG_DATADIR, mask_files, landmarks_file = cedalion.datasets.get_colin27_segmentation()
PARCEL_DIR = cedalion.datasets.get_colin27_parcel_file()
elif HEAD_MODEL == "icbm152":
SEG_DATADIR, mask_files, landmarks_file = cedalion.datasets.get_icbm152_segmentation()
PARCEL_DIR = cedalion.datasets.get_icbm152_parcel_file()
else:
raise ValueError("unknown head model")
The segmentation masks are in individual niftii files. The dict mask_files
maps mask filenames relative to SEG_DATADIR
to short labels. These labels describe the tissue type of the mask.
In principle the user is free to choose these labels. However, they are later used to lookup the tissue’s optical properties. So they must be map to one of the tabulated tissue types (c.f. cedalion.imagereco.tissue_properties.TISSUE_LABELS
).
The variable landmarks_file
holds the path to a file containing landmark positions in scanner space (RAS). This file can be created with Slicer3D.
[13]:
display(SEG_DATADIR)
display(mask_files)
display(landmarks_file)
'/home/runner/.cache/cedalion/v25.1.0/colin27_segmentation.zip.unzip/colin27_segmentation'
{'csf': 'mask_csf.nii',
'gm': 'mask_gray.nii',
'scalp': 'mask_skin.nii',
'skull': 'mask_bone.nii',
'wm': 'mask_white.nii'}
'landmarks.mrk.json'
Coordinate systems
Up to now we have geometrical data from three different coordinate reference systems (CRS):
The optode positions are in one space
CRS='digitized'
and the coordinates are in meter. In our example the origin is at the head center and y-axis pointing in the superior direction. Other digitization tools can use other units or coordinate systems.The segmentation masks are in voxel space (
CRS='ijk'
) in which the voxel edges are aligned with the coordinate axes. Each voxel has unit edge length, i.e. coordinates are dimensionless. Axis-aligned grids are computationally efficient, which is why the photon simulation code (MCX) uses this coordinate system.The voxel space (
CRS='ijk'
) is related to scanner space (CRS='ras'
orCRS='aligned'
) in which coordinates have physical units and coordinate axes point to the (r)ight, (a)nterior and s(uperior) directions. The relation between both spaces is given through an affine transformation (e.g.t_ijk2ras
). When loading the segmentation masks in Slicer3D this transformation is automatically applied. Hence, the picked landmark coordinates are exported in RAS space.The niftii file provides a string label for the scanner space. In this example the RAS space is called ‘aligned’ because the masks are aligned to another MRI scan.
To avoid confusion between these different coordinate systems, cedalion
tries to be explicit about which CRS a given point cloud or surface is in.
The TwoSurfaceHeadModel
The photon propagation considers the complete MRI scan, in which each voxel is attributed to one tissue type with its respective optical properties. However, the image reconstruction does not intend to reconstruct absorption changes in each voxel. The inverse problem is simplified, by considering only two surfaces (scalp and brain) and reconstruct only absorption changes in voxels close to these surfaces.
The class cedalion.imagereco.forward_model.TwoSurfaceHeadModel
groups together the segmentation mask, landmark positions and affine transformations as well as the scalp and brain surfaces. The brain surface is calculated by grouping together white and gray matter masks. The scalp surface encloses the whole head.
[14]:
head = fw.TwoSurfaceHeadModel.from_surfaces(
segmentation_dir=SEG_DATADIR,
mask_files = mask_files,
brain_surface_file= os.path.join(SEG_DATADIR, "mask_brain.obj"),
scalp_surface_file= os.path.join(SEG_DATADIR, "mask_scalp.obj"),
landmarks_ras_file=landmarks_file,
parcel_file=PARCEL_DIR,
brain_face_count=None,
scalp_face_count=None
)
[15]:
head.segmentation_masks
[15]:
<xarray.DataArray (segmentation_type: 5, i: 181, j: 217, k: 181)> Size: 36MB 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 ... 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 Coordinates: * segmentation_type (segmentation_type) <U5 100B 'csf' 'gm' ... 'skull' 'wm' Dimensions without coordinates: i, j, k
[16]:
head.landmarks
[16]:
<xarray.DataArray (label: 4, ijk: 3)> Size: 96B [] 89.95 205.8 35.86 91.76 25.05 17.14 18.05 109.9 17.34 165.9 113.0 17.92 Coordinates: * label (label) <U3 48B 'Nz' 'Iz' 'LPA' 'RPA' type (label) object 32B PointType.LANDMARK ... PointType.LANDMARK Dimensions without coordinates: ijk
[17]:
head.brain
[17]:
TrimeshSurface(mesh=<trimesh.Trimesh(vertices.shape=(15002, 3), faces.shape=(29988, 3))>, crs='ijk', units=<Unit('dimensionless')>, vertex_coords={'parcel': array(['VisCent_ExStr_8_LH', 'VisCent_ExStr_8_LH', 'VisCent_Striate_2_LH',
..., 'SalVentAttnA_ParMed_6_RH', 'ContA_PFCl_5_RH',
'Background+FreeSurfer_Defined_Medial_Wall_RH'],
shape=(15002,), dtype='<U44')})
[18]:
head.scalp
[18]:
TrimeshSurface(mesh=<trimesh.Trimesh(vertices.shape=(10050, 3), faces.shape=(20096, 3))>, crs='ijk', units=<Unit('dimensionless')>, vertex_coords={})
TwoSurfaceHeadModel.from_segmentation
converts everything into voxel space (CRS='ijk'
)
[19]:
head.crs
[19]:
'ijk'
The transformation matrix to translate from voxel to scanner space:
[20]:
head.t_ijk2ras
[20]:
<xarray.DataArray (aligned: 4, ijk: 4)> Size: 128B [mm] 1.0 0.0 0.0 -90.0 0.0 1.0 0.0 -126.0 0.0 0.0 1.0 -72.0 0.0 0.0 0.0 1.0 Dimensions without coordinates: aligned, ijk
Changing between coordinate systems:
[21]:
head_ras = head.apply_transform(head.t_ijk2ras)
display(head_ras.crs)
display(head_ras.brain)
'aligned'
TrimeshSurface(mesh=<trimesh.Trimesh(vertices.shape=(15002, 3), faces.shape=(29988, 3))>, crs='aligned', units=<Unit('millimeter')>, vertex_coords={'parcel': array(['VisCent_ExStr_8_LH', 'VisCent_ExStr_8_LH', 'VisCent_Striate_2_LH',
..., 'SalVentAttnA_ParMed_6_RH', 'ContA_PFCl_5_RH',
'Background+FreeSurfer_Defined_Medial_Wall_RH'],
shape=(15002,), dtype='<U44')})
Optode Registration
The optode coordinates from the recording must be aligned with the scalp surface. Currently, cedaĺion
offers a simple registration method, which finds an affine transformation (scaling, rotating, translating) that matches the landmark positions of the head model and their digitized counter parts. Afterwards, optodes are snapped to the nearest vertex on the scalp.
[22]:
geo3d_snapped_ijk = head.align_and_snap_to_scalp(geo3d_meas)
display(geo3d_snapped_ijk)
<xarray.DataArray (label: 346, ijk: 3)> Size: 8kB [] 11.61 128.0 90.59 22.65 134.1 125.5 ... 172.7 138.4 33.95 173.3 127.9 33.21 Coordinates: type (label) object 3kB PointType.SOURCE ... PointType.LANDMARK * label (label) <U6 8kB 'S1' 'S2' 'S3' 'S4' ... 'FFT10h' 'FT10h' 'FTT10h' Dimensions without coordinates: ijk
[23]:
plt = pv.Plotter()
cedalion.plots.plot_surface(plt, head.brain, color="w")
cedalion.plots.plot_surface(plt, head.scalp, opacity=.1)
cedalion.plots.plot_labeled_points(plt, geo3d_snapped_ijk)
plt.show()
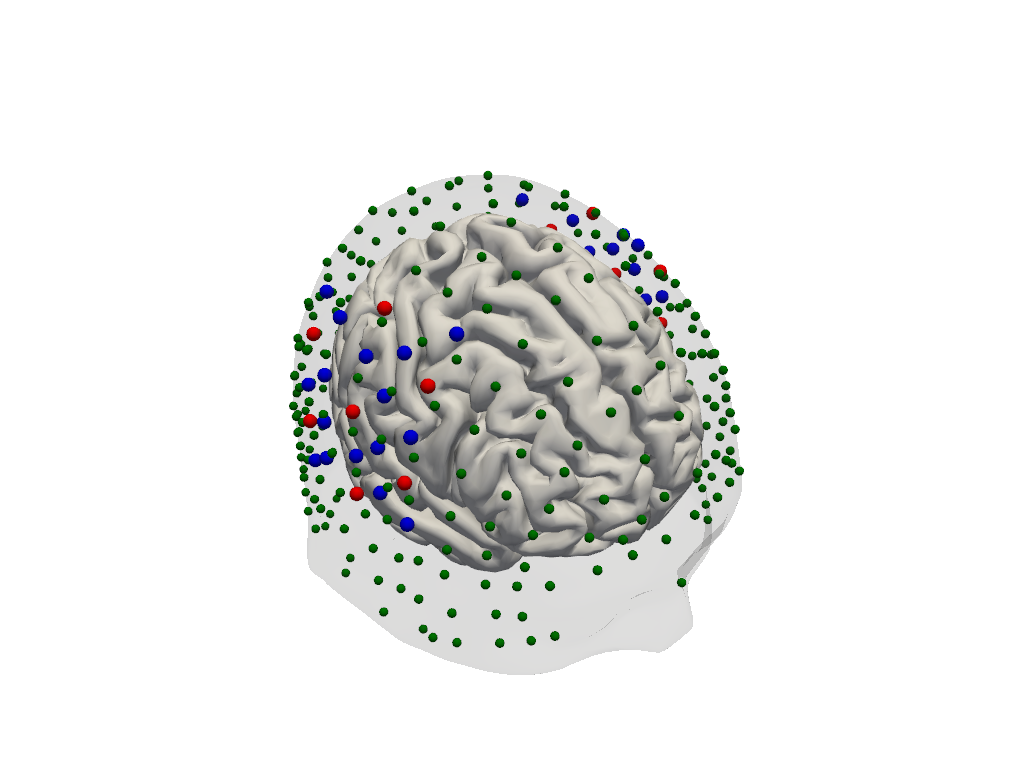
Simulate light propagation in tissue
cedalion.imagereco.forward_model.ForwardModel
is a wrapper around pmcx. Using the data in the head model it prepares the inputs for either pmcx or NIRFASTer and offers functionality to calculate the sensitivty matrix.
[24]:
fwm = cedalion.imagereco.forward_model.ForwardModel(head, geo3d_snapped_ijk, meas_list)
Run the simulation
The compute_fluence_mcx
and compute_fluence_nirfaster
methods simulate a light source at each optode position and calculate the fluence in each voxel. By setting RUN_PACKAGE
, you can choose between the pmcx or NIRFASTer package to perform this simulation. PLEASE NOTE: if you USE_CACHED data (download the example data) be aware that the file is quite big (~2GB).
[25]:
if PRECOMPUTED_FLUENCE:
if FORWARD_MODEL == "MCX":
fluence_fname = cedalion.datasets.get_precomputed_fluence(DATASET, HEAD_MODEL)
elif FORWARD_MODEL == "NIRFASTER":
raise NotImplementedError(
"Currently there are no precomputed NIRFASTER results available"
)
else:
fluence_fname = working_directory / "fluence.h5"
if FORWARD_MODEL == "MCX":
fwm.compute_fluence_mcx(fluence_fname)
elif FORWARD_MODEL == "NIRFASTER":
fwm.compute_fluence_nirfaster(fluence_fname)
The photon simulation yields the fluence in each voxel for each wavelength:
fluence_all
is axr.DataArray
with dimensions: (‘label’, ‘wavelength’, ‘i’, ‘j’, ‘k’),i.e. for each optode and wavelength it stores the 3D image of the computed fluence in each voxel
fluence_at_optodes
is axr.DataArray
with dimensions: (‘optode1’, ‘optode2’, ‘wavelength’).It contains the fluence directly at the position of the optodes, used for normalization purposes.
Both arrays are stored on disk in the hdf5 file at fluence_fname
and should be queried through cedalion.io.forward_model.FluenceFile
.
Also, for a each combination of two optodes, the fluence in the voxels at the optode positions is calculated.
Plot fluence
To illustrate the tissue probed by light travelling from a source to the detector two fluence profiles need to be multiplied.
[26]:
# for plotting use a geo3d without the landmarks
geo3d_plot = geo3d_snapped_ijk[geo3d_snapped_ijk.type != cdc.PointType.LANDMARK]
[27]:
time.sleep(1)
plt = pv.Plotter()
if DATASET == "fingertappingDOT":
src, det, wl = "S5", "D16", 760
elif DATASET == "fingertapping":
src, det, wl = "S2", "D3", 760
else:
raise ValueError("unknown dataset")
# fluence_file.get_fluence returns a 3D numpy array with the fluence
# for a specified source and wavelength.
with FluenceFile(fluence_fname) as fluence_file:
f = fluence_file.get_fluence(src, wl) * fluence_file.get_fluence(det, wl)
f[f <= 0] = f[f > 0].min()
f = np.log10(f)
vf = pv.wrap(f)
plt.add_volume(
vf,
log_scale=False,
cmap="plasma_r",
clim=(-10, 0),
)
cedalion.plots.plot_surface(plt, head.brain, color="w")
cedalion.plots.plot_labeled_points(plt, geo3d_plot, show_labels=False)
cog = head.brain.vertices.mean("label").values
plt.camera.position = cog + [-300, 30, 100]
plt.camera.focal_point = cog
plt.camera.up = [0, 0, 1]
plt.show()
/home/runner/miniconda3/envs/cedalion/lib/python3.11/site-packages/xarray/core/variable.py:315: UnitStrippedWarning: The unit of the quantity is stripped when downcasting to ndarray.
data = np.asarray(data)
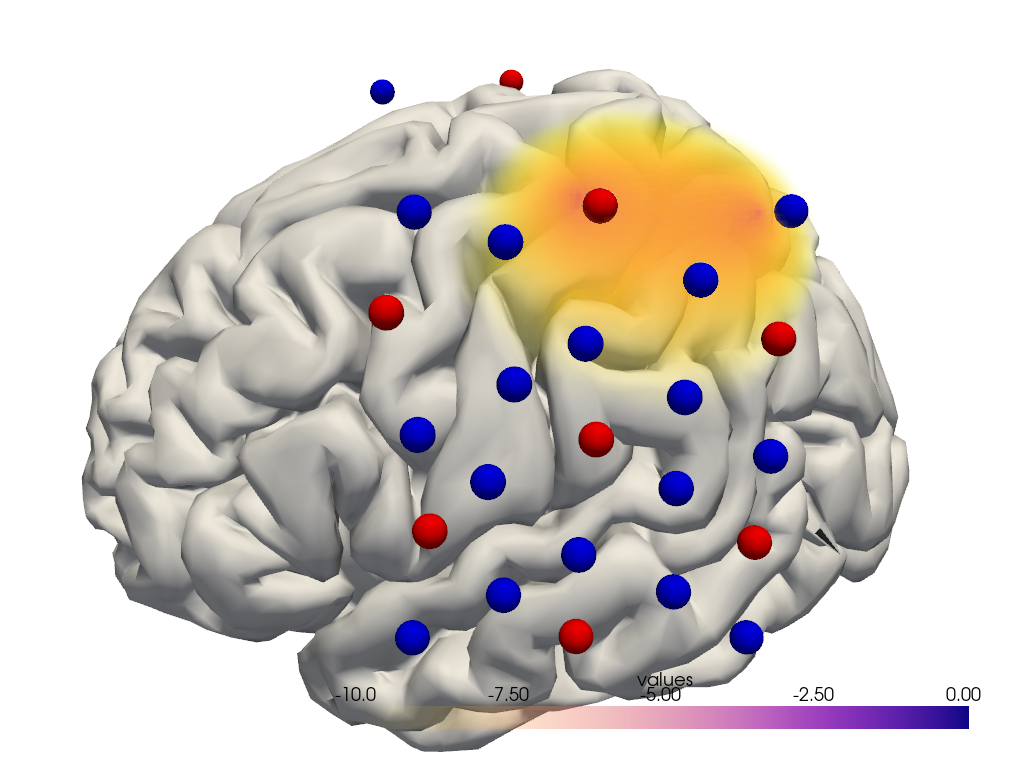
head.brain.vertices
Calculate the sensitivity matrices
The sensitivity matrix describes the effect of an absorption change at a given surface vertex in the OD recording in a given channel and at given wavelength. The coordinate is_brain
holds a mask to distinguish brain and scalp voxels.
[28]:
sensitivity_fname = working_directory / "sensitivity.h5"
fwm.compute_sensitivity(fluence_fname, sensitivity_fname)
[29]:
Adot = load_Adot(sensitivity_fname)
Plot Sensitivity Matrix
[30]:
plotter = cedalion.vis.plot_sensitivity_matrix.Main(
sensitivity=Adot,
brain_surface=head.brain,
head_surface=head.scalp,
labeled_points=geo3d_plot,
)
plotter.plot(high_th=0, low_th=-3)
plotter.plt.show()
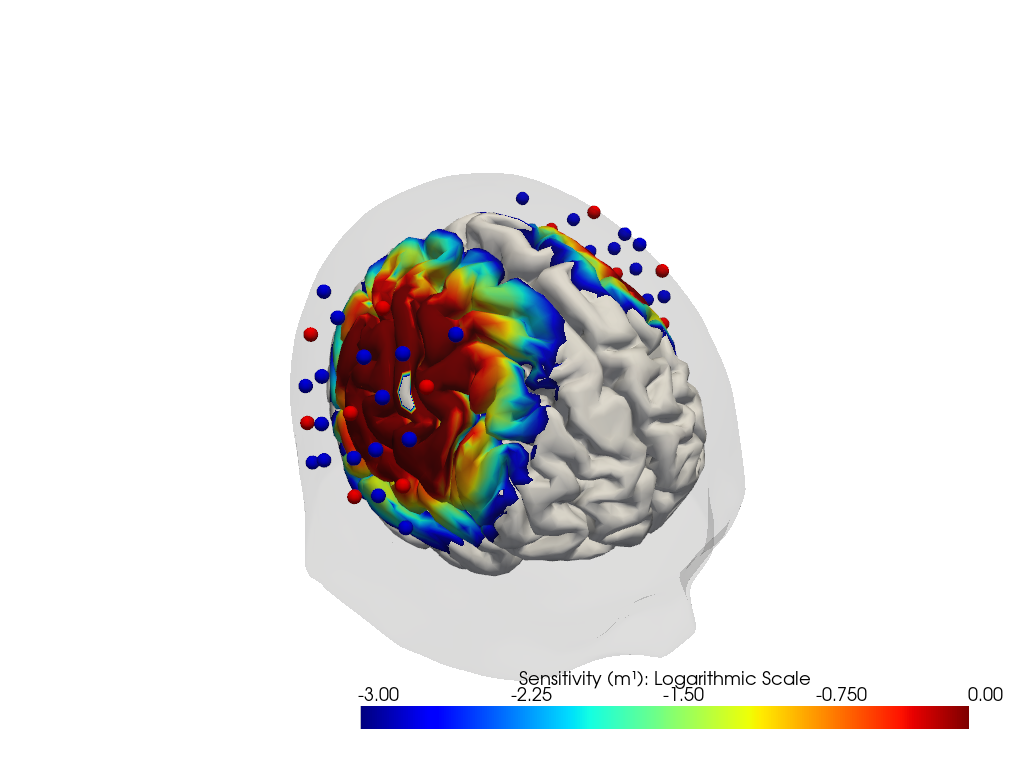
The sensitivity Adot
has shape (nchannel, nvertex, nwavelenghts). To solve the inverse problem we need a matrix that relates OD in channel space to absorption in image space. Hence, the sensitivity must include the extinction coefficients to translate between OD and concentrations. Furthermore, channels at different wavelengths must be stacked as well vertice and chromophores into new dimensions (flat_channel, flat_vertex):
[31]:
Adot_stacked = fwm.compute_stacked_sensitivity(Adot)
Adot_stacked
[31]:
<xarray.DataArray (flat_channel: 200, flat_vertex: 50104)> Size: 80MB 1.635e-15 5.346e-18 5.545e-17 7.044e-18 ... 0.0 4.463e-18 3.12e-16 6.072e-14 Coordinates: is_brain (flat_vertex) bool 50kB True True True ... False False False chromo (flat_vertex) <U3 601kB 'HbO' 'HbO' 'HbO' ... 'HbR' 'HbR' 'HbR' vertex (flat_vertex) int64 401kB 0 1 2 3 4 ... 25048 25049 25050 25051 wavelength (flat_channel) float64 2kB 760.0 760.0 760.0 ... 850.0 850.0 channel (flat_channel) object 2kB 'S1D1' 'S1D2' ... 'S14D31' 'S14D32' source (flat_channel) object 2kB 'S1' 'S1' 'S1' ... 'S14' 'S14' 'S14' detector (flat_channel) object 2kB 'D1' 'D2' 'D4' ... 'D29' 'D31' 'D32' parcel (flat_vertex) object 401kB 'VisCent_ExStr_8_LH' ... 'scalp' Dimensions without coordinates: flat_channel, flat_vertex Attributes: units: 1 / molar
Invert the sensitivity matrix
[32]:
B = pseudo_inverse_stacked(Adot_stacked, alpha = 0.01, alpha_spatial = 0.001)
B
[32]:
<xarray.DataArray (flat_vertex: 50104, flat_channel: 200)> Size: 80MB 4.742e-17 3.465e-16 -1.81e-16 3.54e-16 ... 5.593e-16 -6.023e-17 6.803e-17 Coordinates: is_brain (flat_vertex) bool 50kB True True True ... False False False chromo (flat_vertex) <U3 601kB 'HbO' 'HbO' 'HbO' ... 'HbR' 'HbR' 'HbR' vertex (flat_vertex) int64 401kB 0 1 2 3 4 ... 25048 25049 25050 25051 wavelength (flat_channel) float64 2kB 760.0 760.0 760.0 ... 850.0 850.0 channel (flat_channel) object 2kB 'S1D1' 'S1D2' ... 'S14D31' 'S14D32' source (flat_channel) object 2kB 'S1' 'S1' 'S1' ... 'S14' 'S14' 'S14' detector (flat_channel) object 2kB 'D1' 'D2' 'D4' ... 'D29' 'D31' 'D32' parcel (flat_vertex) object 401kB 'VisCent_ExStr_8_LH' ... 'scalp' Dimensions without coordinates: flat_vertex, flat_channel Attributes: units: molar
Calculate concentration changes
the optical density has shape (nchannel, nwavelength, time). Additional dimensions like ‘trial_type’ in this example are allowed, too.
[33]:
blockaverage
[33]:
<xarray.DataArray (trial_type: 2, channel: 100, wavelength: 2, reltime: 154)> Size: 493kB [] -0.0006526 -0.001002 -0.001244 -0.00137 ... -0.0003574 -0.0002813 -0.0001787 Coordinates: * reltime (reltime) float64 1kB -5.038 -4.809 -4.58 ... 29.54 29.77 30.0 * channel (channel) object 800B 'S1D1' 'S1D2' 'S1D4' ... 'S14D31' 'S14D32' source (channel) object 800B 'S1' 'S1' 'S1' 'S1' ... 'S14' 'S14' 'S14' detector (channel) object 800B 'D1' 'D2' 'D4' 'D5' ... 'D29' 'D31' 'D32' * wavelength (wavelength) float64 16B 760.0 850.0 * trial_type (trial_type) object 16B 'FTapping/Left' 'FTapping/Right'
To apply the inverted sensitiviy matrix, the OD wavelength and channel dimensions need to be flattened. Then the inverted sensitivity matrix can be multiplied which contracts over flat_channel and the flat_vertex dimension remains. The flat_vertex dimensions containes vertices of the scalp and the brain for both chromophores. These need to be separated again. The function fw.apply_inv_sensitiviy
takes care of all of this.
[34]:
dC_brain, dC_scalp = fw.apply_inv_sensitivity(blockaverage, B)
display(dC_brain)
display(dC_scalp)
<xarray.DataArray (trial_type: 2, reltime: 154, chromo: 2, vertex: 15002)> Size: 74MB -2.502e-16 -2.23e-17 -8.265e-18 -4.766e-17 ... -1.401e-14 5.005e-12 -1.163e-16 Coordinates: * chromo (chromo) <U3 24B 'HbO' 'HbR' * vertex (vertex) int64 120kB 0 1 2 3 4 ... 14997 14998 14999 15000 15001 * reltime (reltime) float64 1kB -5.038 -4.809 -4.58 ... 29.54 29.77 30.0 * trial_type (trial_type) object 16B 'FTapping/Left' 'FTapping/Right' is_brain (chromo, vertex) bool 30kB True True True ... True True True parcel (chromo, vertex) object 240kB 'VisCent_ExStr_8_LH' ... 'Backg... Attributes: units: molar
<xarray.DataArray (trial_type: 2, reltime: 154, chromo: 2, vertex: 10050)> Size: 50MB -1.932e-18 -3.877e-19 -3.965e-18 -5.426e-18 ... 1.683e-19 1.466e-18 6.107e-18 Coordinates: * chromo (chromo) <U3 24B 'HbO' 'HbR' * vertex (vertex) int64 80kB 15002 15003 15004 ... 25049 25050 25051 * reltime (reltime) float64 1kB -5.038 -4.809 -4.58 ... 29.54 29.77 30.0 * trial_type (trial_type) object 16B 'FTapping/Left' 'FTapping/Right' is_brain (chromo, vertex) bool 20kB False False False ... False False parcel (chromo, vertex) object 161kB 'scalp' 'scalp' ... 'scalp' Attributes: units: molar
Convert concentration changes into micromolar.
[35]:
dC_brain = dC_brain.pint.quantify().pint.to("uM").pint.dequantify()
dC_scalp = dC_scalp.pint.quantify().pint.to("uM").pint.dequantify()
Plot concentration changes
Using cedalion plot functions to visualie image recon results
Using Scalp Plot Functionality to Create a Gif of Brain Activity on the Scalp
This gives us the activity in channel space across time.
[36]:
from cedalion.plots import scalp_plot_gif
warnings.filterwarnings("ignore", category=UnitStrippedWarning)
# configure the plot
data_ts = blockaverage.sel(wavelength=850, trial_type="FTapping/Right")
data_ts = data_ts.rename({"reltime": "time"})
geo3d = rec.geo3d
filename_scalp = "scalp_plot_ts"
# call plot function
scalp_plot_gif(
data_ts,
geo3d,
filename=filename_scalp,
time_range=(-5, 30, 0.5) * units.s,
scl=(-0.01, 0.01),
fps=6,
optode_size=6,
optode_labels=True,
str_title="850 nm",
)
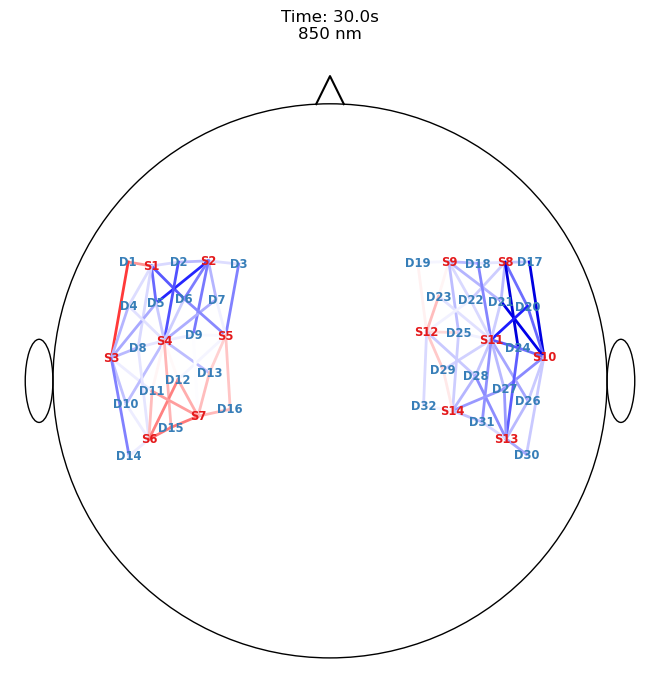
[37]:
display(Image(data=open(filename_scalp+'.gif','rb').read(), format='png'))
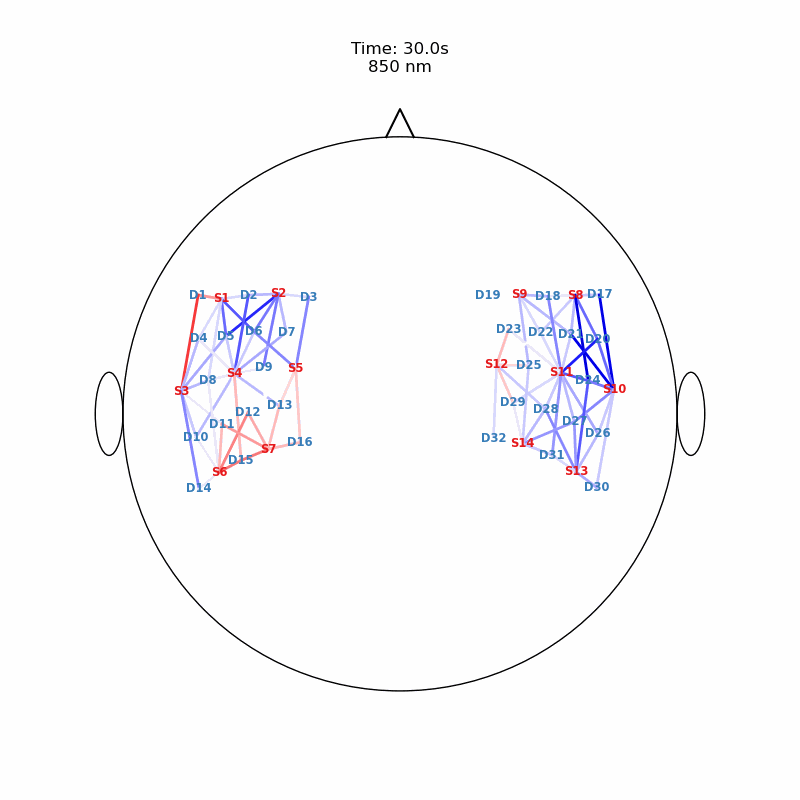
Using Image Recon View Functionality to Create a Gif of Activity on the Brain
This gives us activity on the brain from a single view as still image or across time
[38]:
from cedalion.plots import image_recon_view
filename_view = 'image_recon_view'
X_ts = xr.concat([dC_brain.sel(trial_type="FTapping/Right"), dC_scalp.sel(trial_type="FTapping/Right")], dim="vertex")
X_ts = X_ts.rename({"reltime": "time"})
X_ts = X_ts.transpose("vertex", "chromo", "time")
X_ts = X_ts.assign_coords(is_brain=('vertex', Adot.is_brain.values))
scl = np.percentile(np.abs(X_ts.sel(chromo='HbO').values.reshape(-1)),99)
clim = (-scl,scl)
image_recon_view(
X_ts, # time series data; can be 2D (static) or 3D (dynamic)
head,
cmap='seismic',
clim=clim,
view_type='hbo_brain',
view_position='left',
title_str='HbO / uM',
filename=filename_view,
SAVE=True,
time_range=(-5,30,0.5)*units.s,
fps=6,
geo3d_plot = geo3d_plot,
wdw_size = (1024, 768)
)
[39]:
display(Image(data=open(filename_view+'.gif','rb').read(), format='png'))
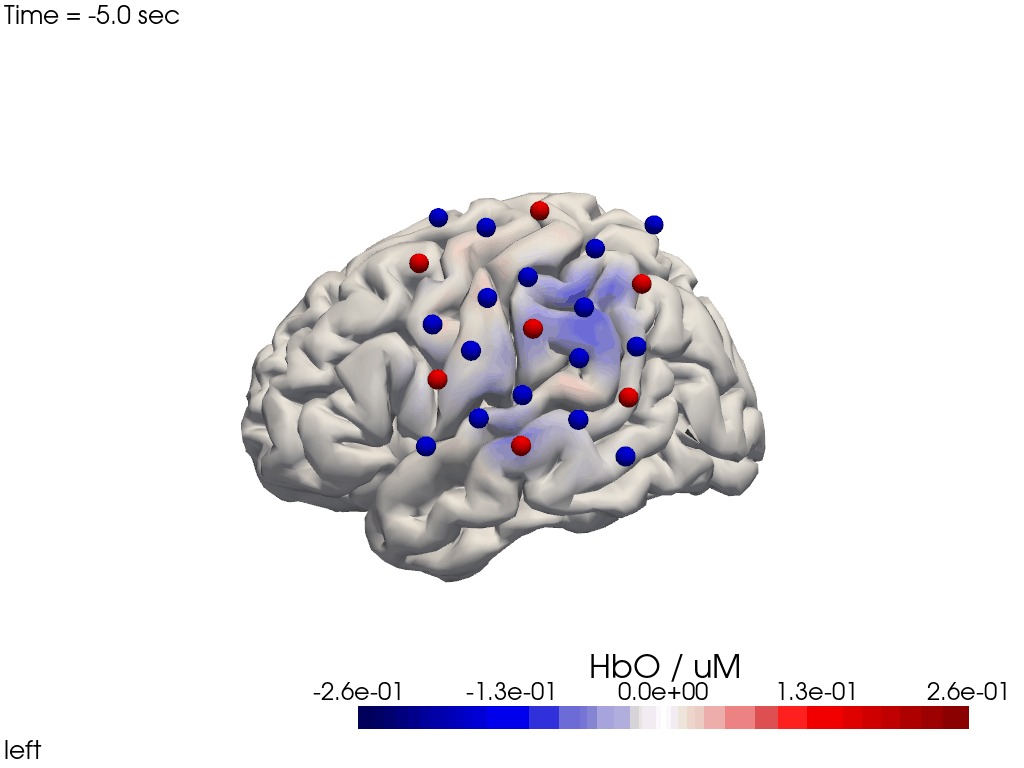
Alternatively, we can just select on time point and plot activity as a still image at that time.
[40]:
# selects the nearest time sample at t=10s in X_ts
X_ts = X_ts.sel(time=4*units.s, method="nearest")
filename_view = 'image_recon_view_still'
image_recon_view(
X_ts, # time series data; can be 2D (static) or 3D (dynamic)
head,
cmap='seismic',
clim=clim,
view_type='hbo_brain',
view_position='left',
title_str='HbO / uM',
filename=filename_view,
SAVE=True,
time_range=(-5,30,0.5)*units.s,
fps=6,
geo3d_plot = geo3d_plot,
wdw_size = (1024, 768)
)
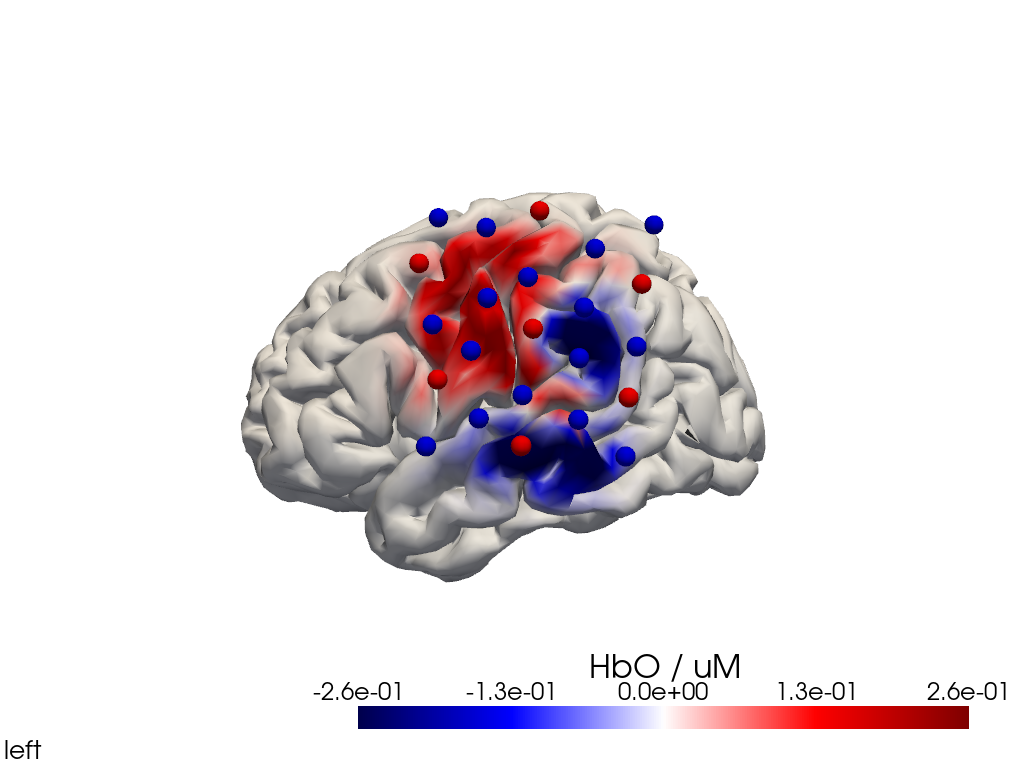
[41]:
display(Image(data=open(filename_view+'.png','rb').read(), format='png'))
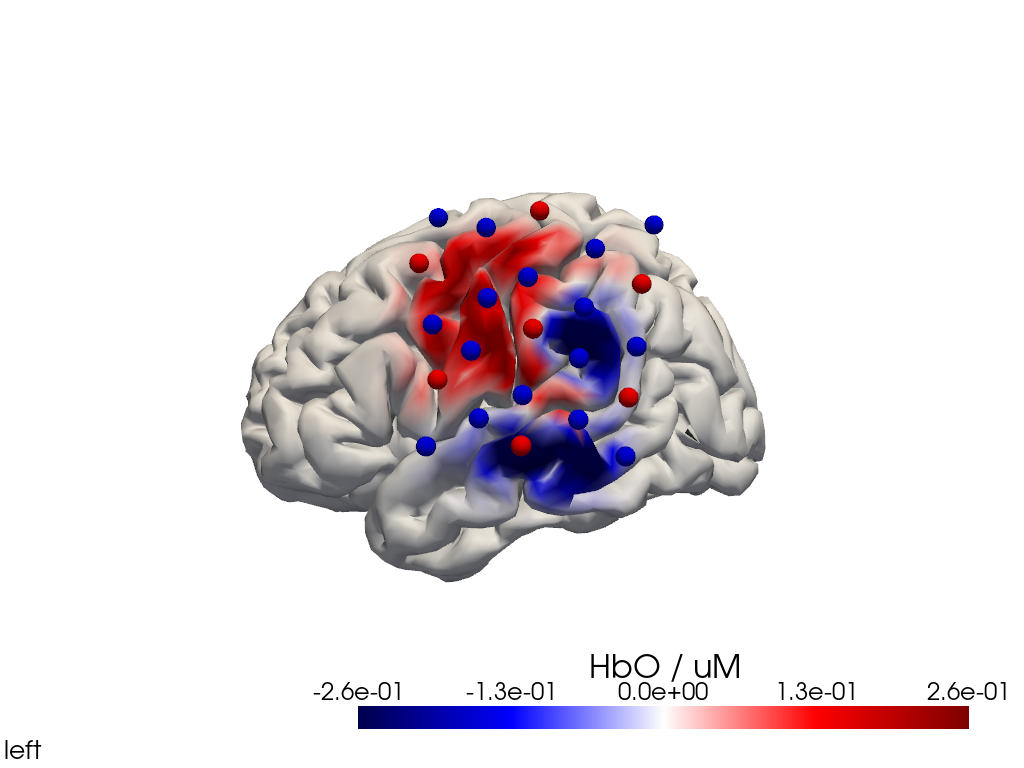
Using Image Recon Multi View Functionality to Create a Gif of Activity on the Brain
This gives us activity on the brain after recon from all angles as still image or across time
[42]:
from cedalion.plots import image_recon_multi_view
filename_multiview = 'image_recon_multiview'
# prepare data
X_ts = xr.concat([dC_brain.sel(trial_type="FTapping/Right"), dC_scalp.sel(trial_type="FTapping/Right")], dim="vertex")
X_ts = X_ts.rename({"reltime": "time"})
X_ts = X_ts.transpose("vertex", "chromo", "time")
X_ts = X_ts.assign_coords(is_brain=('vertex', Adot.is_brain.values))
scl = np.percentile(np.abs(X_ts.sel(chromo='HbO').values.reshape(-1)),99)
clim = (-scl,scl)
image_recon_multi_view(
X_ts, # time series data; can be 2D (static) or 3D (dynamic)
head,
cmap='seismic',
clim=clim,
view_type='hbo_brain',
title_str='HbO / uM',
filename=filename_multiview,
SAVE=True,
time_range=(-5,30,0.5)*units.s,
fps=6,
geo3d_plot = None, # geo3d_plot
wdw_size = (1024, 768)
)
[43]:
display(Image(data=open(filename_multiview+'.gif','rb').read(), format='png'))
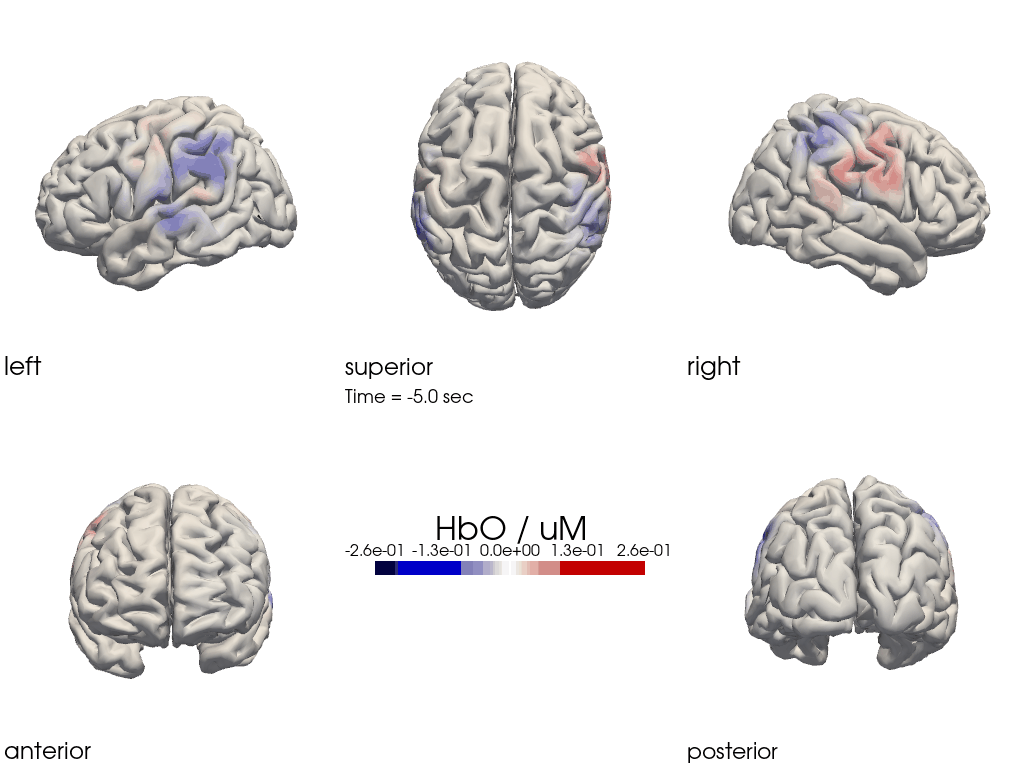
Using Image Recon Multi View Functionality to Create a Gif of Activity on the Scalp
This gives us activity on the scalp after recon from all angles as still image or across time
[44]:
from cedalion.plots import image_recon_multi_view
filename_multiview_scalp = 'image_recon_multiview_scalp'
# prepare data
X_ts = xr.concat([dC_brain.sel(trial_type="FTapping/Right"), dC_scalp.sel(trial_type="FTapping/Right")], dim="vertex")
X_ts = X_ts.rename({"reltime": "time"})
X_ts = X_ts.transpose("vertex", "chromo", "time")
X_ts = X_ts.assign_coords(is_brain=('vertex', Adot.is_brain.values))
scl = np.percentile(np.abs(X_ts.sel(chromo='HbO').values.reshape(-1)),99)
clim = (-scl,scl)
image_recon_multi_view(
X_ts, # time series data; can be 2D (static) or 3D (dynamic)
head,
cmap='seismic',
clim=clim,
view_type='hbo_scalp',
title_str='HbO / uM',
filename=filename_multiview_scalp,
SAVE=True,
time_range=(-5,30,0.5)*units.s,
fps=6,
geo3d_plot = geo3d_plot,
wdw_size = (1024, 768)
)
[45]:
display(Image(data=open(filename_multiview_scalp+'.gif','rb').read(), format='png'))
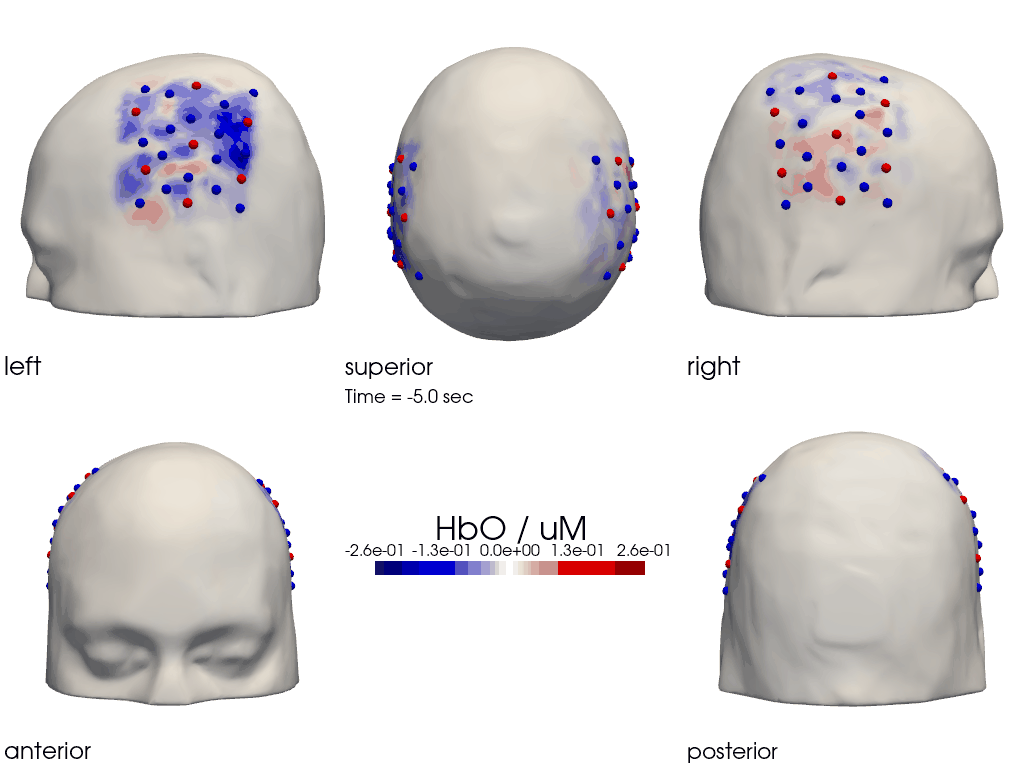
Concentration changes in parcels
We can now calculate concentration changes for each parcellation label. For a specific parcel label, the concentration change is determined by averaging the concentration changes across the corresponding vertices.
[46]:
baseline = dC_brain.sel(reltime=(dC_brain.reltime == dC_brain.reltime[0]))
num_reps = dC_brain.reltime.shape[0]
baseline = [baseline] * num_reps
baseline = xr.concat(baseline, dim='reltime').values
dC_brain = dC_brain - baseline
cells = dC_brain.sel(chromo='HbO').pint.dequantify()
avg_HbO = cells.groupby('parcel').mean()
cells = dC_brain.sel(chromo='HbR').pint.dequantify()
avg_HbR = cells.groupby('parcel').mean()
Plot HbO and HbR
[ ]:
The Schaefer Atlas provides nearly 600 labels, making it impractical to plot concentration changes for all of them. Additionally, since we’re using the Finger Tapping dataset with a limited set of optodes, many brain regions lack significant signal coverage due to the absence of optodes. To focus on relevant regions, we selected a subset of labels based on a criterion: the absolute value of either the minimum or maximum concentration change must exceed 0.01. Using this method, we identified 60
parcellations as active_labels
.
[47]:
selected_parcels = ["SomMotA_4_LH", "SomMotA_9_LH", "SomMotA_5_LH", "SomMotA_6_LH",
"SomMotA_7_LH", "SomMotA_8_LH",
"SomMotA_4_RH", "SomMotA_9_RH", "SomMotA_5_RH", "SomMotA_6_RH",
"SomMotA_7_RH", "SomMotA_8_RH"]
[48]:
f,ax = p.subplots(2,6, figsize=(20,5))
ax = ax.flatten()
for i_par, par in enumerate(selected_parcels):
ax[i_par].plot(avg_HbO.sel(parcel = par, trial_type = "FTapping/Right").reltime, avg_HbO.sel(parcel = par, trial_type = "FTapping/Right").values, "r", lw=2, ls='-')
ax[i_par].plot(avg_HbR.sel(parcel = par, trial_type = "FTapping/Right").reltime, avg_HbR.sel(parcel = par, trial_type = "FTapping/Right").values, "b", lw=2, ls='-')
ax[i_par].grid(1)
ax[i_par].set_title(par)
ax[i_par].set_ylim(-.05, .2)
p.suptitle("Parcellations: HbO: r | HbR: b | right: -", y=1)
p.tight_layout()
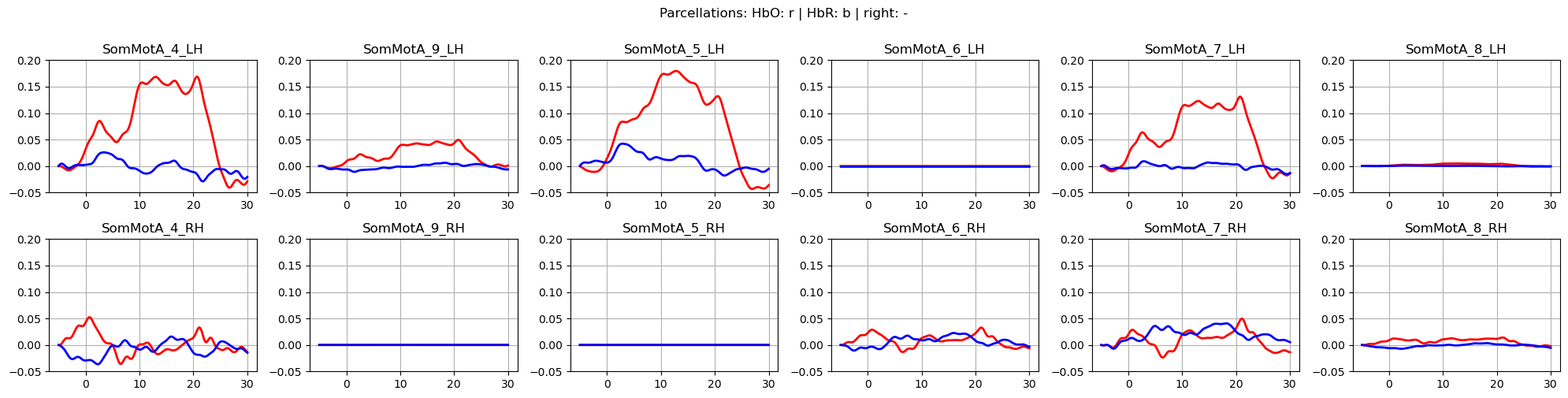